YAML - YAML ain't Markup language
SDL - Simple Declarative Language
JSON - JavaScript Object Notation
XML - Extensible Markup Language
YAML
It is a human friendly data serialization standard for all programming languages. It is specially suited for tasks where humans are likely to view or edit data structures such as configuration files, log files, inter-process messaging, cross-language data sharing.
It uses unicode printable characters to show data in natural and more meaningful way.
JSON ‘n’ YAML have different priorities. JSON’s foremost design goal is simplicity ‘n’ universality. Thus, JSON is trivial to generate and parse at the cost of reduced human readability. In contrast, YAML’s foremost design goals are human readability and support for serializing arbitrary native data structures. Thus, YAML allows for extremely readable files, but is more complex to generate and parse. YAML is viewed as superset of JSON and hence every JSON file is also a valid YAML file.
Below is a snippet of how a yaml file looks like:
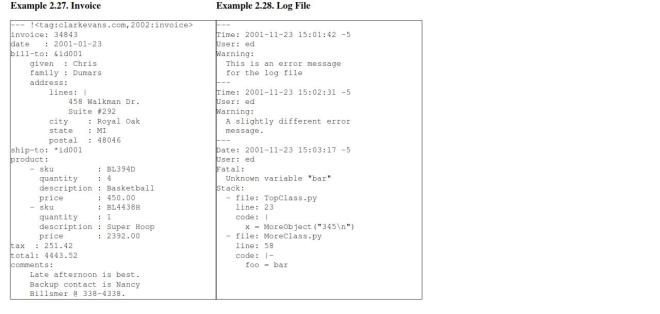
SnakeYAML is a YAML parser and emitter for java programming language.
SDL
If two systems need to exchange data, the easiest way to do this is to pass the data as text since most systems can parse and process a textual input. The format of the text (indenting, keywords, special characters) is used to describe the data being exchanged. SDL is one more such format.
The Simple Declarative Language provides an easy way to describe lists, maps, and trees of typed data in a compact, easy to read representation. The simple and intuitive API allows you to read, write, and access all the datastructures using a single class. For property files, configuration files, logs, and simple serialization requirements, SDL provides a compelling alternative to XML and Properties files. Implementations are available for Java and .NET with more languages on the way.
Note: SDL homepage is down! Seems no new developments happening here!
people location="Tokyo" {
person "Akiko" friendly=true {
hobbies {
hobby "hiking" times_per_week=2
hobby "swimming" times_per_week=1
}
}
person "Jim" {
hobbies {
hobby "karate" times_per_week=5
}
}
}
JSON
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. These properties make JSON an ideal data-interchange language.
JSON is built on two structures:
- Object: An object is an unordered set of name-value pairs. An object begins with { (left brace) and ends with } (right brace). Each name is followed by : (colon) and the name/value pairs are separated by , (comma).

- Array: An array is an ordered collection of values. An array begins with [ (left bracket) and ends with ] (right bracket). Values are separated by , (comma).

A value can be a string in double quotes, or a number, or true or false or null, or an object or an array. These structures can be nested.

Following is an XML representation of some data:
<menu id="file" value="File">
<popup>
<menuitem value="New" onclick="CreateNewDoc()" />
<menuitem value="Open" onclick="OpenDoc()" />
<menuitem value="Close" onclick="CloseDoc()" />
</popup>
</menu>
and below is it’s corresponding JSON representation:
{"menu": {
"id": "file",
"value": "File",
"popup": {
"menuitem": [
{"value": "New", "onclick": "CreateNewDoc()"},
{"value": "Open", "onclick": "OpenDoc()"},
{"value": "Close", "onclick": "CloseDoc()"}
]
}
}}
A java implementation of JSON is JSON-lib.
Add the following dependency in pom.xml file of your maven project to get JSON-lib jars.
<dependency>
<groupId>net.sf.json-lib</groupId>
<artifactId>json-lib</artifactId>
<version>2.4</version>
</dependency>
XML
XML was created to structure, store, and transport information. It is just information wrapped in tags. Someone must write a piece of software to send, receive or display it.
<?xml version="1.0"?>
<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend!</body>
</note>
It is widely used for the representation of arbitrary data structures (mostly in web services).
Existing APIs for XML processing:
- SAX: Document is read serially and its contents are reported as callbacks to various methods on a handler object of the user’s design. SAX is fast and efficient to implement, but difficult to use for extracting information at random from the XML, since it tends to burden the application author with keeping track of what part of the document is being processed.
- DOM: Document Object Model is an interface oriented API that allows for navigation of the entire document as if it were a tree of node objects representing the document’s contents. DOM implementations tend to be memory intensive, as they generally require the entire document to be loaded into memory and constructed as a tree of objects before access is allowed.